简介
FragmentTabHost是一种特殊的TabHost,允许我们在Tab内容区域内使用Fragment。当我们得到FragmentTabHost对象后,我们必须通过调用setup(Context, FragmentManager, int)方法来完成对tabhost的初始化。
FragmentTabHost是Android4.0版本的控件, 之前被广泛的使用,但是我尽然没有使用过,因为我开始学习Android的时候版本已经来到了5.0版本,使用的比较多的是TabLayout+ViewPager这种方式。但是作为基础知识,FragmentTabHost也是需要学习一下的。
类结构
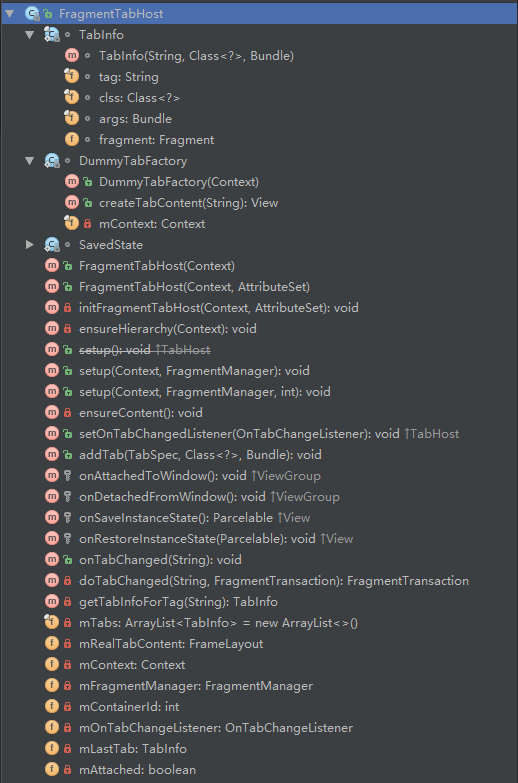
FragmentTabHost继承TabHost。先看一下public方法
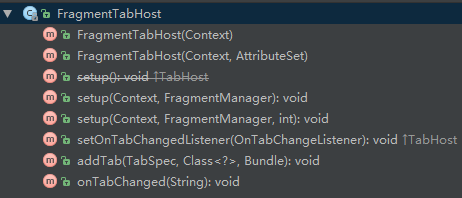
方法 |
描述 |
public FragmentTabHost(Context context) |
构造方法 |
public FragmentTabHost(Context context, AttributeSet attrs) |
构造方法 |
public void setup(Context context, FragmentManager manager)/public void setup(Context context, FragmentManager manager, int containerId) |
FragmentTabHost初始化方法,必须调用此方法完成初始化 |
public void setOnTabChangedListener(OnTabChangeListener l) |
设置页面切换监听器 |
public void addTab(@NonNull TabHost.TabSpec tabSpec, @NonNull Class<?> clss,@Nullable Bundle args) |
添加标签 |
public void onTabChanged(String tabId) |
FragmentTabHost实现TabHost.OnTabChangeListener |
基本使用
布局文件
activity_main.xml
注意:
android:id=”@android:id/tabhost”
android:id=”@android:id/tabcontent”
android:id=”@android:id/tabs”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <?xml version="1.0" encoding="utf-8"?> <android.support.v4.app.FragmentTabHost android:id="@android:id/tabhost" xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent">
<LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <FrameLayout android:id="@android:id/tabcontent" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1"/> <TabWidget android:id="@android:id/tabs" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:layout_gravity="bottom"/> </LinearLayout>
</android.support.v4.app.FragmentTabHost>
|
fragment_content.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical">
<TextView android:id="@+id/tab_tv_text" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:textSize="40sp" tools:text="Test"/>
</LinearLayout>
|
indicator.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <ImageView android:id="@+id/iv_indicator" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="5"/>
<TextView android:id="@+id/tv_indicator" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="2.5" android:gravity="center" android:textSize="5pt" /> </LinearLayout>
|
代码
MainActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| public class MainActivity extends AppCompatActivity { @DrawableRes private int mImages[] = { R.drawable.tab_daily, R.drawable.tab_sort, R.drawable.tab_bonus, R.drawable.tab_about };
private String mFragmentTags[] = { "每日干货", "分类阅读", "福利社区", "关于作者" }; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
FragmentTabHost fragmentTabHost = (FragmentTabHost) this.findViewById(android.R.id.tabhost); fragmentTabHost.setup(this,getSupportFragmentManager(), android.R.id.tabcontent);
for (int i = 0; i < mImages.length; i++) { TabHost.TabSpec tabSpec = fragmentTabHost.newTabSpec(mFragmentTags[i]).setIndicator(getImageView(i)); fragmentTabHost.addTab(tabSpec, YFragment.class, null); fragmentTabHost.getTabWidget().getChildAt(i).setBackgroundResource(R.color.colorWhite); }
fragmentTabHost.setOnTabChangedListener(new TabHost.OnTabChangeListener() { @Override public void onTabChanged(String s) { Toast.makeText(MainActivity.this, "OnTabChanged To : " + s, Toast.LENGTH_SHORT).show(); } });
}
private View getImageView(int index) { @SuppressLint("InflateParams") View view = getLayoutInflater().inflate(R.layout.indicator, null); ImageView imageView = view.findViewById(R.id.iv_indicator); TextView textView = view.findViewById(R.id.tv_indicator); imageView.setImageResource(mImages[index]); textView.setText(mFragmentTags[index]); return view; } }
|
YFragment.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public class YFragment extends Fragment {
TextView mTvText;
@Nullable @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { View view = inflater.inflate(R.layout.fragment_content, container, false); mTvText = view.findViewById(R.id.tab_tv_text); return view; }
@Override public void onViewCreated(View view, @Nullable Bundle savedInstanceState) { super.onViewCreated(view, savedInstanceState); mTvText.setText(String.valueOf(getTag())); }
}
|
图片资源
tab_daily.xml
1 2 3 4 5
| <?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_selected="true" android:drawable="@drawable/tab_daily_select"/> <item android:drawable="@drawable/tab_daily_unselect"/> </selector>
|
其余几个tab标签的内容参照如上
效果
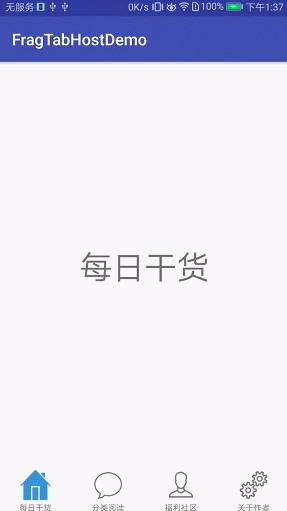
其他测试
activity_main.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <?xml version="1.0" encoding="utf-8"?> <android.support.v4.app.FragmentTabHost android:id="@android:id/tabhost" xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent">
<LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <FrameLayout android:id="@android:id/tabcontent" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="1"/> </LinearLayout>
</android.support.v4.app.FragmentTabHost>
|
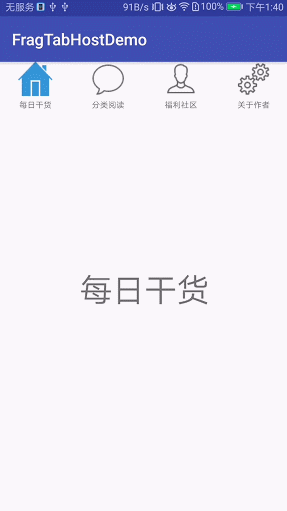
操作一:注释掉Id为@android:id/tabcontent的内容
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <?xml version="1.0" encoding="utf-8"?> <android.support.v4.app.FragmentTabHost android:id="@android:id/tabhost" xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent">
<LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> </LinearLayout>
</android.support.v4.app.FragmentTabHost>
|
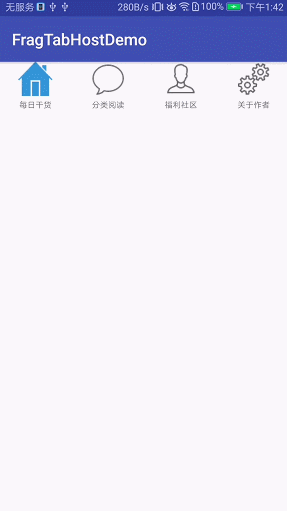
备注
第五部分的解释可以从FragmentTabHost的源码中分析得到。